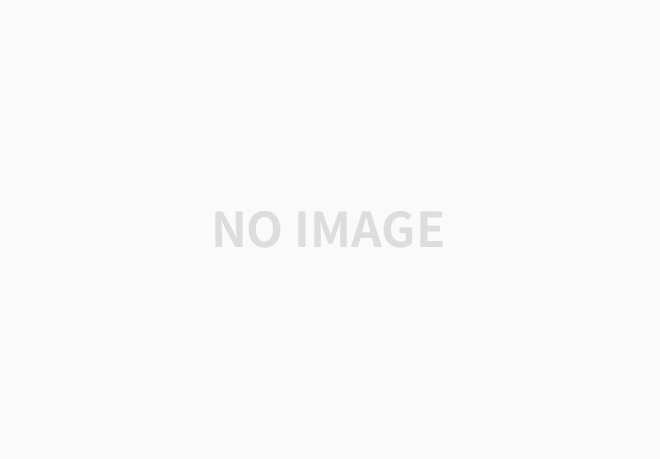
withRouter는 라우터 컴포넌트가 아닌 곳에서 match, location, history를 props로 받아와서 사용하게 해줍니다.
src/WithRouterSample.js
import React from 'react';
import { withRouter } from 'react-router-dom';
const WithRouterSample = ({ location, match, history }) => {
return (
<div>
<h4>location</h4>
<textarea value={JSON.stringify(location, null, 2)} readOnly />
<h4>match</h4>
<textarea value={JSON.stringify(match, null, 2)} readOnly />
<button onClick={() => history.push('/')}>홈으로</button>
</div>
);
};
export default withRouter(WithRouterSample);
Profiles.js 에서 렌더링하기
src/Profiles.js
import React from "react";
import Profile from "./Profile";
import { Link, Route } from "react-router-dom";
import WithRouterSample from './WithRouterSample';
function Profiles() {
return (
<div>
<h3>사용자 목록</h3>
<ul>
<li>
<Link to="/profiles/meanbymin">meanbymin</Link>
</li>
<li>
<Link to="/profiles/homer">homer</Link>
</li>
</ul>
<Route
exact
path="/profiles"
render={() => <div>사용자를 선택해주세요</div>}
/>
<Route path="/profiles/:username" component={Profile} />
<WithRouterSample />
</div>
);
}
export default Profiles;
withRouter를 사용하면, match같은 경우는 현재 자신이 렌더링 된 위치를 기준으로 match값을 받아옵니다. 그렇다면 결국에 match에서 사용하는 값은 profiles에서 받아오는 값이랑 동일한데, profiles에서는 받아오는 값이 없기때문에 match params에는 {} 빈 형태가 나옵니다. 그렇기 때문에 profiles 파일에서 렌더링 하는 것이 아니라 profile에서 렌더링을 해주어야합니다.
src/Profiles.js에서 import WithRouterSample from './WithRouterSample'; 와 <WithRouterSample />을 삭제해주세요.
src/Profile.js
import React from "react";
import WithRouterSample from "./WithRouterSample";
const profileData = {
meanbymin: {
name: "오석민",
description: "Frontend Engineer @sangnam",
},
homer: {
name: "호머 심슨",
description: "심슨 가족에 나오는 아빠역할 캐릭터",
},
};
function Profile({ match }) {
const { username } = match.params;
const profile = profileData[username];
if (!profile) return <div>존재하지 않는 사용자입니다.</div>;
return (
<div>
<h3>
{username} ({profile.name})
</h3>
<p>{profile.description}</p>
<WithRouterSample />
</div>
);
}
export default Profile;
profile 컴포넌트에서 WithRouterSample에서 렌더링하면 profile에서 match를 받아오기때문에 params에 정보가 잘 나타나는 것을 볼 수 있습니다.
✔️ location같은 경우에는 어디에서 불러오든 똑같은 정보를 가리키고 있는데, match같은 경우는 WithRouterSample을 렌더링하는 곳의 정보를 가져온다는 것을 기억해주시면 좋습니다.
💡 withRouter는 보통 어떤 용도로 사용하냐면, route로 사용되지 않은 컴포넌트에서 조건부로 이동해야할 때 사용합니다. 예시로 로그인 성공하였을 때 특정 경로로 가고 실패하였을 때 페이지를 유지하려고 할 때 사용합니다.
'Front-End > React' 카테고리의 다른 글
[React] Redux란 ? (0) | 2021.06.04 |
---|---|
[React] react-router-dom switch, NavLink (0) | 2021.06.04 |
[React] react-route-dom history (0) | 2021.06.03 |
[React] 서브라우트 (0) | 2021.06.03 |
[React] Router에서 파라미터와 쿼리 (0) | 2021.06.03 |
댓글